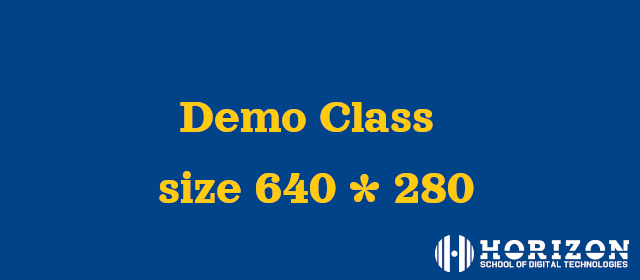
This course is a Template, please add your description and change the course photo.
- Teacher: Ahmed Jalali
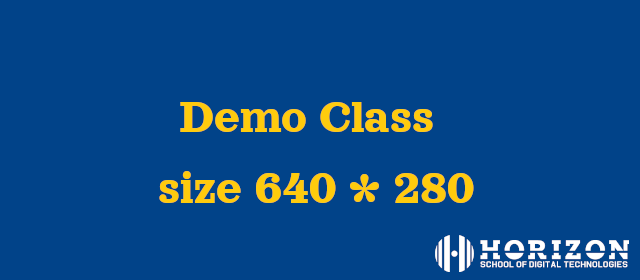
This course is a Template, please add your description and change the course photo.
- Teacher: Malek Ben Salem
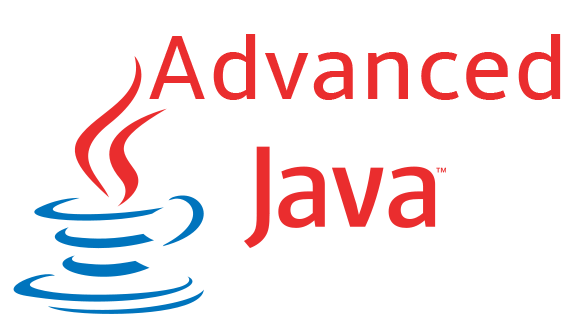
Advanced Java Course Summary
In this Advanced Java course, students will deepen their understanding of Java programming, covering both foundational and advanced concepts essential for building robust, scalable applications. The course is structured to blend theoretical knowledge with hands-on experience in building real-world backend applications.
Course Topics:
-
Java Language Basics:
- Review of fundamental Java syntax, data types, and control structures.
- Focus on refining coding practices and enhancing problem-solving skills in Java.
-
Object-Oriented Programming (OOP):
- Classes and Objects: Deep dive into defining and using classes, constructors, and instance variables.
- Inheritance: Understanding and implementing class hierarchies, extending classes, and method overriding.
- Polymorphism: Exploring compile-time and runtime polymorphism for flexible, reusable code.
- Encapsulation: Ensuring data integrity through private fields and public getter/setter methods.
- Abstraction: Using abstract classes and interfaces to define common contracts and decouple implementation from interface.
-
Java Collections Framework:
- Detailed exploration of core data structures: List, Set, Map, and Queue.
- Understanding the differences between common implementations (ArrayList, LinkedList, HashMap, TreeSet, etc.).
- Best practices for selecting the appropriate collection based on use case requirements.
-
Java Streams API:
- Introduction to Streams for processing sequences of data.
- Stream operations: filter, map, reduce, collect, and sort.
- How to leverage functional programming features in Java with lambda expressions.
-
Concurrency in Java:
- Introduction to multi-threading and concurrency concepts.
- Managing threads using ExecutorService, synchronization, and locks.
- Best practices for avoiding race conditions and deadlocks.
-
Functional Programming in Java:
- Understanding and applying functional programming techniques in Java.
- Lambda expressions, method references, and functional interfaces.
- Introduction to the Optional class for safer null handling.
-
Advanced Java Features:
- Java 8 and beyond: Exploring new language features, such as default methods in interfaces, streams, and optional.
- Java Modules: Organizing large applications using the Java Platform Module System (JPMS).
-
Spring Boot (Optional, time permitting):
- Introduction to Spring Boot framework for building microservices and backend REST APIs.
- RESTful Web Services: Implementing endpoints using Spring MVC and handling HTTP requests/responses.
- Database Integration: Using Spring Data JPA for connecting with relational databases.
- Security: Basic authentication and authorization with Spring Security.
- Testing: Writing unit and integration tests using JUnit and MockMvc.
-
Building a Backend REST Application:
- Hands-on project where students apply all concepts learned to build a real-world RESTful API with Spring Boot.
- Emphasis on clean code, design patterns, and best practices for backend development.
Course Objectives:
By the end of the course, students will:
- Gain a strong command over advanced Java concepts.
- Be proficient in Java collections, streams, and functional programming techniques.
- Understand object-oriented principles and design patterns for scalable application development.
- Have hands-on experience building a backend API with Spring Boot (if time allows).
This course is designed to provide students with the skills needed to become proficient Java developers capable of tackling complex software development challenges and building efficient, maintainable applications.
- Teacher: Mohamed Amine Marzouk
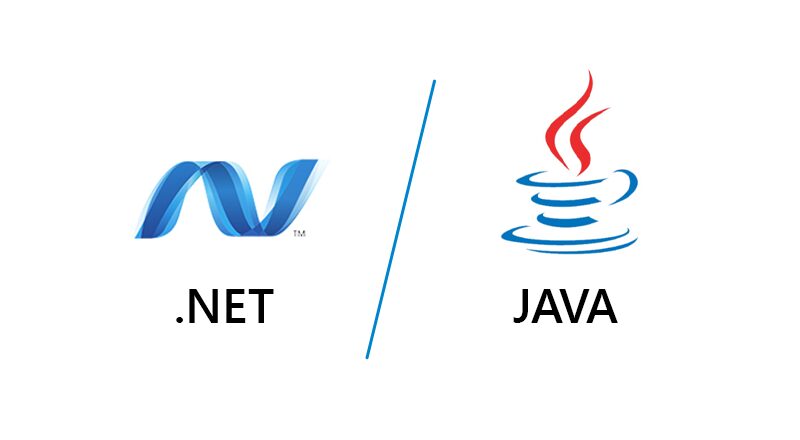
Middleware and Frameworks (JEE/.NET) Course Summary
The Middleware and Frameworks (JEE/.NET) course provides students with a comprehensive understanding of enterprise application development, focusing on two of the most widely used frameworks: Java EE (now Jakarta EE) and .NET Core. The course balances theoretical concepts with hands-on labs and practical projects, enabling students to build real-world enterprise applications.
Course Topics:
- Introduction to Enterprise Applications:
- Overview of enterprise application development: What constitutes an enterprise application, and the challenges associated with building scalable, maintainable, and secure systems.
- Key architectural patterns used in enterprise apps (e.g., layered architecture, MVC, Microservices, SOA).
- Understanding the role of middleware in enterprise systems: server-side technologies that handle communication, data processing, and integration across multiple systems.
- Introduction to Enterprise Integration Patterns (EIP) and how they are applied to Java EE and .NET environments.
- Java EE (Jakarta EE) Basics:
- Overview of Java EE: Key components of Java EE (now Jakarta EE), and how they enable the development of large-scale, multi-tiered, and distributed applications.
- Servlets and JSP: Introduction to Java web applications, handling HTTP requests, and creating dynamic web pages.
- Enterprise JavaBeans (EJB): Using EJBs for business logic and transaction management in enterprise applications.
- JPA (Java Persistence API): Managing relational data with JPA, ORM (Object-Relational Mapping) concepts, and integrating with relational databases.
- JMS (Java Message Service): Message-driven applications and integration using JMS for asynchronous communication.
- Web Services: Building SOAP and RESTful web services with JAX-RS (Java API for RESTful Web Services).
- Security: Implementing authentication and authorization in Java EE applications using JAAS (Java Authentication and Authorization Service).
- Hands-on exercises in building a multi-tiered enterprise application using JSP, Servlets, EJB, and JPA.
- Developing a RESTful web service using JAX-RS and consuming it with a frontend client.
-
.NET Core Basics:
- Introduction to .NET Core: Overview of the cross-platform, open-source .NET framework. How it differs from traditional .NET Framework and its role in building modern enterprise applications.
- ASP.NET Core: Building web applications using MVC (Model-View-Controller) architecture with ASP.NET Core MVC.
- Entity Framework Core: ORM framework for data access. Using EF Core for database integration and management.
- Dependency Injection: Implementing Dependency Injection in .NET Core to decouple components and improve testability and maintainability.
- Web API: Building and consuming RESTful APIs using ASP.NET Core Web API.
- Authentication & Authorization: Managing authentication and securing APIs with ASP.NET Core Identity and JWT (JSON Web Tokens) for token-based authentication.
- Microservices Architecture: Introduction to Microservices with ASP.NET Core, focusing on building small, loosely coupled services that work together in a larger system.
- Testing: Writing unit and integration tests for .NET Core applications using xUnit and Moq.
Labs & Practical Projects:
- Hands-on projects where students create a complete web application using ASP.NET Core MVC and connect it to a relational database with Entity Framework Core.
- Building a RESTful API with ASP.NET Core Web API and securing it using JWT.
- Developing a Microservices-based application in ASP.NET Core.
- Integration and Middleware Concepts:
- Middleware in Java EE and .NET Core: Understanding the role of middleware in web application frameworks (e.g., handling requests, processing data, security checks).
- Message Queues: Using JMS in Java EE and Azure Service Bus or RabbitMQ in .NET Core to handle message-based communication between services.
- API Gateway and Service Discovery: Implementing API gateways in Spring Cloud (for Java) and Ocelot (for .NET Core) for routing, load balancing, and security.
- Caching and Performance Optimization: Techniques for improving performance using caching strategies (e.g., EHCache in Java and Redis in .NET Core).
- Final Project:
- Students will apply what they've learned by working on a final project that involves building an enterprise-level application combining both Java EE (Jakarta EE) and .NET Core technologies.
- The project will require designing a microservices architecture, integrating databases, implementing security, and deploying the application for scalability.
Course Objectives:
By the end of this course, students will:
- Gain a deep understanding of enterprise-level application development.
- Be proficient in Java EE (Jakarta EE) and .NET Core frameworks for building scalable, secure, and maintainable applications.
- Understand key architectural patterns (e.g., MVC, Microservices) and how to implement them in both Java and .NET ecosystems.
- Be able to build full-stack applications using Java EE (Servlets, EJB, JPA, JMS) and ASP.NET Core (MVC, Web API, EF Core, Dependency Injection).
- Have practical experience working on real-world enterprise applications, including integration, security, and performance optimization.
This course prepares students to tackle enterprise development challenges using two powerful and widely adopted frameworks. It equips them with the knowledge and skills needed to develop, deploy, and maintain large-scale enterprise applications in both Java and .NET ecosystems.
- Teacher: Mohamed Amine Marzouk
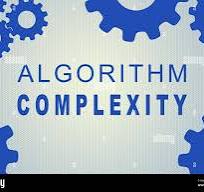
This course is a Template, please add your description and change the course photo.
- Teacher: Rania Yangui
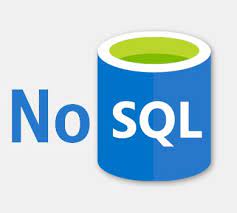
This course is a Template, please add your description and change the course photo.
- Teacher: Rania Yangui
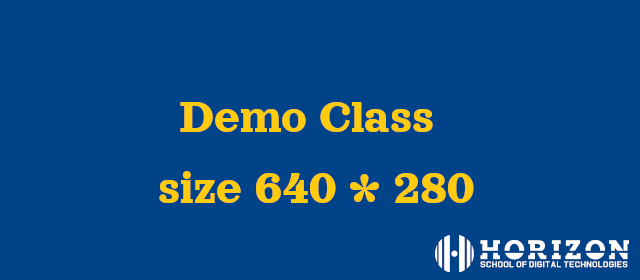
This course is a Template, please add your description and change the course photo.
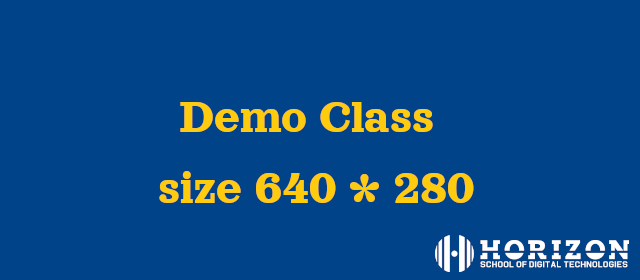
This course is a Template, please add your description and change the course photo.
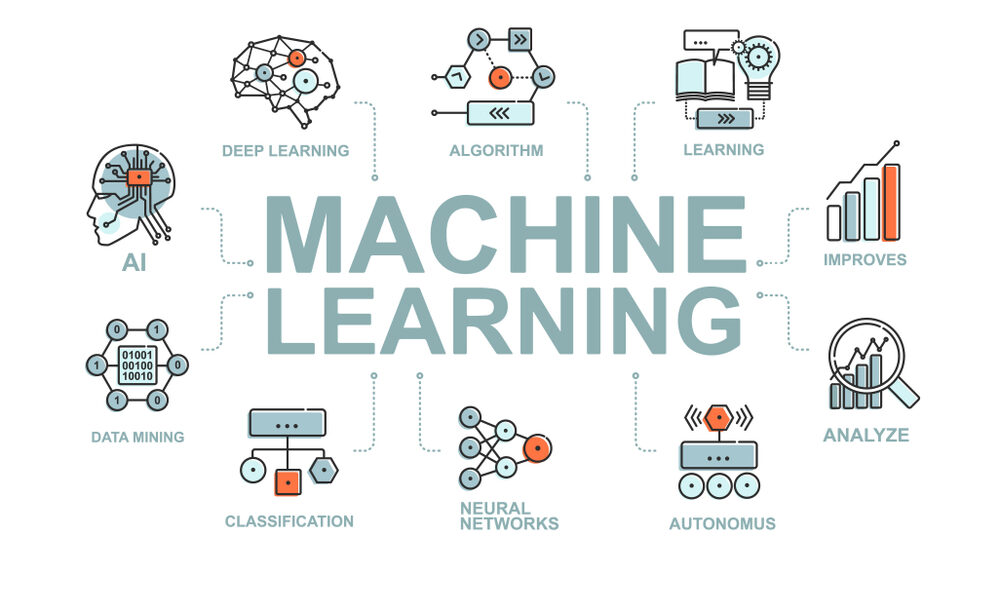