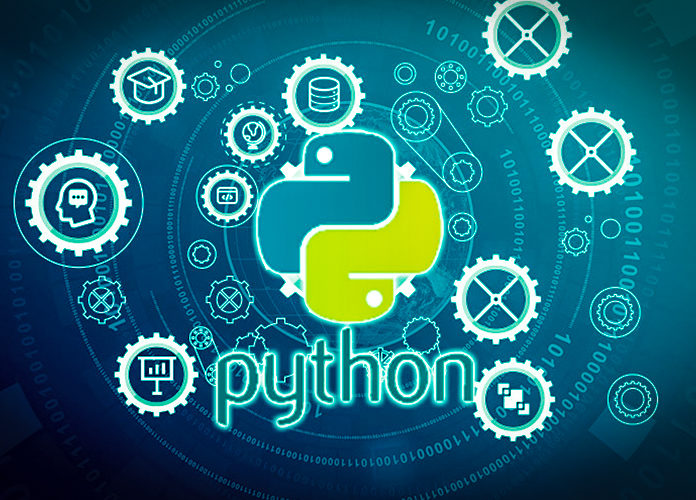
Course Description:
Welcome to the Python Programming course! This course is designed to introduce you to the fundamentals of programming using Python, one of the most popular and versatile programming languages in the world. Whether you're a complete beginner or have some prior experience, this course will equip you with the skills to write efficient, readable, and well-structured Python code.
Throughout this course, you will learn the core concepts of Python, including data types, control structures, functions, and object-oriented programming. You will also explore how to work with files, handle errors, and use Python libraries to solve real-world problems. By the end of the course, you will have the confidence to build your own Python projects and continue your journey as a programmer.
Course Objectives:
By the end of this course, you will be able to:
-
Understand and apply Python syntax and programming concepts.
-
Write, debug, and optimize Python programs.
-
Use data structures like lists, tuples, dictionaries, and sets effectively.
-
Implement control structures such as loops and conditional statements.
-
Create and use functions to modularize code.
-
Understand the basics of object-oriented programming (OOP) in Python.
-
Work with files and handle exceptions in your programs.
-
Utilize Python libraries and modules to extend functionality.
-
Develop simple projects to solve real-world problems.
Course Outline:
-
Introduction to Python
-
What is Python?
-
Setting up the Python environment (IDEs, Jupyter Notebook, etc.)
-
Writing and running your first Python program
-
-
Python Basics
-
Variables and data types
-
Input and output operations
-
Basic operators and expressions
-
-
Control Structures
-
Conditional statements (if, elif, else)
-
Loops (for, while)
-
Break and continue statements
-
-
Data Structures
-
Lists, tuples, sets, and dictionaries
-
List comprehensions
-
Iterating through data structures
-
-
Functions
-
Defining and calling functions
-
Function arguments and return values
-
Lambda functions
-
-
Object-Oriented Programming (OOP)
-
Classes and objects
-
Inheritance and polymorphism
-
Encapsulation and abstraction
-
-
File Handling and Exception Handling
-
Reading from and writing to files
-
Handling exceptions using try-except blocks
-
-
Python Libraries and Modules
-
Introduction to popular libraries (e.g., NumPy, Pandas, Matplotlib)
-
Importing and using modules
-
-
Final Project
-
Apply what you've learned to build a small Python project
-
- Teacher: Mohamed Amine Marzouk